Bubble Sorting algorithm and Program in C#
Bubble sort is a technique to sort an unordered array (or collection) into a sorted one. Bubble sort is a common algorithm and can be used in any programming language. Bubble sort can be used to arrange the data either in ascending order or descending order.
Here is the Selection Sorting Algorithm and Program in C#.
How Bubble Sort Works
The main idea behind the bubble sort is to compare the two adjacent elements and swap them if necessary.
Let’s say we have the following array. And we want to sort this array using the bubble sort in C#.
int[] arr = { 12, 10, 8, 1, 25 };
Iteration 1
In the first iteration (Assuming we are arranging the data in ascending order)
- We will start by comparing the first two elements (
arr[0] & arr[1]
). - If the
arr[0]
is large than thearr[1]
then we will swap them or if thearr[0]
is already smaller than thearr[1]
then there is no need to do anything and we will leave it as it is. - We will follow this process for
arr.Length - 1
times. - At the end of the first iteration, the largest element (12 in our case) will move to the end of the array.
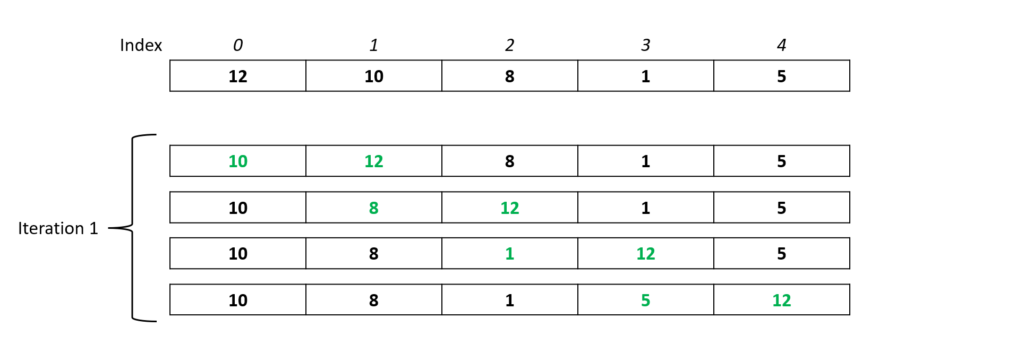
Just have a look on the above figure of first iteration. If we talk little bit technical here then we have to run a loop from
0 to arr.Length-1
.And Because the largest element is already at the end (after this first iteration), so ideally in the next iteration we should run the loop from
0 to arr.Lenth-1-1
.
Let’s see what will happen in Iteration 2.
Iteration 2
In the second iteration again we will follow the same step but this time we will run the loop till arr.Length-1-1
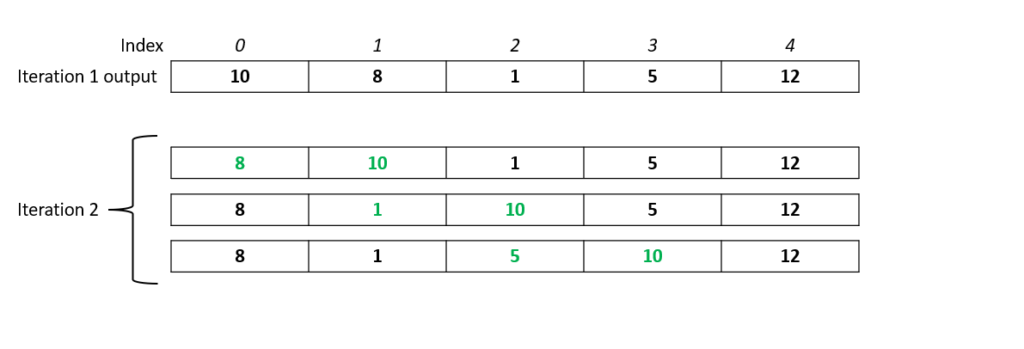
Now after two iterations the last two elements are already sorted. If you are following it carefully, in the next iteration we will need to run our loop from 0 to arr.Length-1-1-1
i.e arr.Length-1-2
. Let’s do it.
Iteration 3
Here is the iteration 3 image
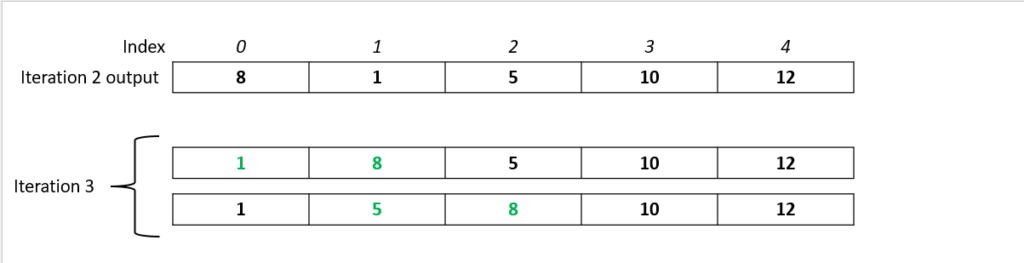
Just focus: Last 3 elements are sorted.
Iteration 4
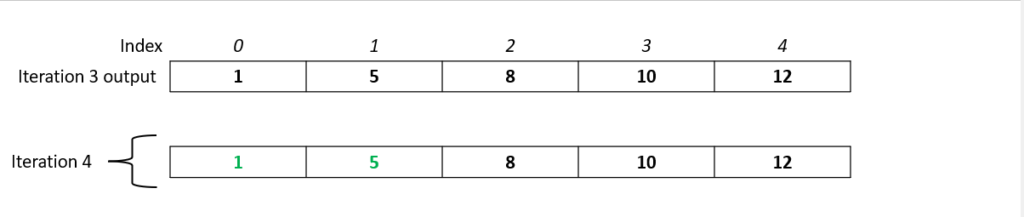
Wow, You did it.
That was the last iteration of this bubble sort. And after this iteration, the data is sorted in ascending order.
Note: You may have fewer or more iterations based on the length of your array. Total iterations = array.Length-1
Convert this bubble sort algorithm to a C# program
Think before writing the code.
Question: How many loops are required?
Ans: 2.
One loop (Outer) will be used for the number of iterations which is from 0 to arr.Length-1.
The second loop (Inner) will be used to compare the two adjacent elements and we will swap the data if required. But it will go from 0 to arr.Length-1-i
Here is the C# program for bubble sorting in ascending order
public static int[] BubbleSort(int[] array)
{
for (int i = 0; i < array.Length - 1; i++)
{
for (int j = 0; j < array.Length - 1 - i; j++)
{
if (array[j] > array[j + 1])
{
(array[j], array[j + 1]) = (array[j + 1], array[j]);
}
}
}
return array;
}
I am assuming the loop logic is clear now let’s talk about the swapping concept.
In line number 7 we are comparing the adjacent elements. If the first one is larger than the second one then we will swap them.
In line number 8 we are swapping the values using the tuple concept in C#. You can also use the traditional way means using a third variable or without using a third variable.
Bubble sort algorithm for descending order in C#
I want you to implement this logic by yourself because we have already explained the entire logic of bubble sort in detail. (Please read this post again).
But if you are in hurry and just need the code. Here is how to write a bubble sort program in descending order using C#.
You have to replace only one condition at line number 7 from (array[j] > array[j + 1])
to if (array[j] < array[j + 1])
.
Thank you for reading. I hope it was helpful.