View Components in Asp.Net Core with an example – [ Ultimate guide ]
What are view components in asp.net core? Why should we use web components in asp.net core? What is the difference between web components and partial views?
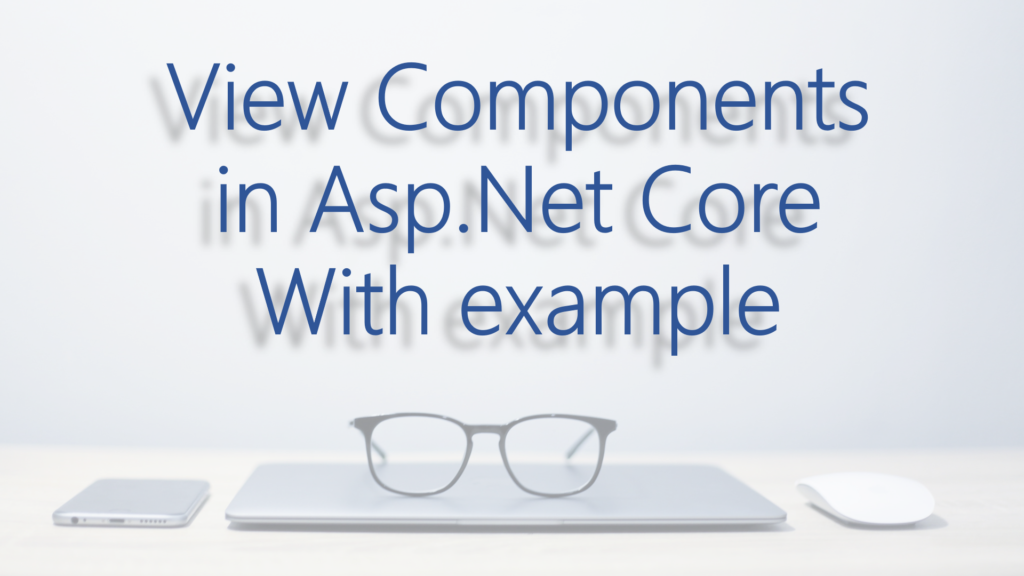
If you are an asp.net core developer or you are still learning about asp.net core, then you must have heard all the above-written questions frequently.
Well, Today in this blog post I will explain the actual concept of view components in asp.net core.
What are the view components in asp.net core?
ViewComponent is a special feature that is used to render some data (view + data) on a view file without actually being the part of the complete Http life cycle.
These are one of the main building blocks and used internally in asp.net core. View components are used to render response but this is not a whole response rather it is just a chunk.
What features can we create using view components?
View components are developed to serve a very important role in asp.net core framework. View components can be used to create the following features:
- Dynamic navigation menu (Based on role etc)
- Get some related data for a page. (Like: Related post, related books)
- Shopping cart
- Any content visible on the side of web page
- etc.
What all files are available in a view component?
A view component typically consist of minimum 2 files in asp.net core.
- Server side file (.cs file).
- Client side file (.cshtml file)
What should be the location for the files of view components?
In asp.net core there is a special place for the view component’s file.
Server side file (.cs) –
This file can be created anywhere in the solution. But we generally create a new folder (with name Components
, ViewComponents
or any other name as per your choice) at the root level of the project and put all the view components in this new folder.
Client side file (.cshtml) –
The client side file of a view component must be placed at a specific location.
- If we want to call the view component from the
controller's action method
then we need to add the view component client-side file at the following location-
/Views/{Controller Name}/Components/{View Component Name}/{View Name}
- If we want to call the view component from the other
cshtml
page then we need to add the view component client-side file at the following location-
/Views/Shared/Components/{View Component Name}/{View Name}
- If we are using a view component in
Razor pages
then we need to add the view component client-side file at the following location-
/Pages/Shared/Components/{View Component Name}/{View Name}
The name for each view component file should be
You can also have some other names for your view component client-side file. But the recommended one is Default.cshtmlDefault.cshtml
How to invoke a view component on view file in asp.net core mvc-
We can invoke the view component from a view file by using following code –
@await Component.InvokeAsync("Name of view component", {Anonymous Type Containing Parameters});
How to invoke a view component on view file using Tag helper in asp.net core mvc-
View component can also be invoked using tag helper on any view (cshtml) file.
<vc:[view-component-name]
parameter1="parameter1 value"
parameter2="parameter2 value">
</vc:[view-component-name]>
Example of View component in Asp.net core –
Lets say we want to display some top books on a particular page of Asp.Net Core MVC application by using view component then we need to perform following actions.
Repository file-
The code of getting top books from the database is written in BooksRepository.cshtml
file.
public class BookRepository
{
public async Task<List<BookModel>> GetTopBooksAsync(int count)
{
return await _context.Books
.Select(book => new BookModel()
{
Author = book.Author,
Category = book.Category,
Description = book.Description,
Id = book.Id,
LanguageId = book.LanguageId,
Language = book.Language.Name,
Title = book.Title,
TotalPages = book.TotalPages,
CoverImageUrl = book.CoverImageUrl
}).Take(count).ToListAsync();
}
}
You can have less or more properties in your BookModel
class.
View Component server-side file –
Now, We need to create the view component server-side file. We can add the server-side file at any location (let’s say /components
folder) in the project.
Suppose the name of view component server-side file is TopBooks
then we must add a suffix ViewComponent
to its name. Hence the final name of the view compone t server-side file will be – TopBooksViewComponent
public class TopBooksViewComponent : ViewComponent
{
/// <summary>
/// Book repository private field
/// </summary>
private readonly BookRepository _bookRepository;
/// <summary>
/// Constructor having a constructor dependency for BookRepository
/// </summary>
/// <param name="bookRepository"></param>
public TopBooksViewComponent(BookRepository bookRepository)
{
_bookRepository = bookRepository;
}
/// <summary>
/// The Invoje method for View component
/// </summary>
/// <param name="count"></param>
/// <returns></returns>
public async Task<IViewComponentResult> InvokeAsync(int count)
{
var books = await _bookRepository.GetTopBooksAsync(count);
return View(books);
}
}
View Component client-side file –
Since we are using asp.net core mvc and invoking this view component from a view file hence we need to place the client side file at following location –
/Views/Shared/Components/TopBooks/Default.cshtml
@model IEnumerable<BookModel>
<div class="row">
@foreach (var book in Model)
{
<div class="col-md-4">
<div class="card-body">
<h3 class="card-title">@book.Title</h3>
<p class="card-text">@book.Description</p>
<div class="d-flex justify-content-between align-items-center">
<div class="btn-group">
<a asp-route="bookDetailsRoute" asp-route-id="@book.Id"
class="btn btn-sm btn-outline-secondary">View
details</a>
</div>
<small class="text-muted">@book.Author</small>
</div>
</div>
</div>
}
</div>
Our view component is completed and ready to use.
1. Now we just need to invoke it from another view file.
@await Component.InvokeAsync("TopBooks", new { count = 3})
Here TopBooks
is the name of view component and count
is the parameter with its value 3
. You can assign any value to count
parameter.
2. Now we just need to invoke it from another view file using Tag helper.
<vc:top-books count="3" ></vc:top-books>
Note: We need to translate Pascal-cased class and method parameters into their kebab case
Result:
And finally you will see all the top books on your UI.
You can also learn about View Component from following video –
I hope this will be helpful to you. Thank you 🙂