Modern C#: Practice programs for Console Class in CSharp.
There is only one way to learn any programming. And that only way is Practice. This post has 5 programs to practice the Console Class in C#.
Please create the following programs to practice the console window concept in the C# programming language.
Note: Try to create the program by yourself without any external help. The solution of each program is given below in the same post but refer to the solution only to match your program or if you are stuck.
Create a C# program to display your full name on the console window.
Output: My name is your name goes here
Create another C# program to display all the day’s names of the week.
Output:Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Create a C# program that allows the user to enter his/her 5 family member names and display them one by one on the console window.
Output:Enter the first name
The user will enter the value here
Your first member is ..........
Enter the second name
The user will enter the value here
Your second member is ..........
display all five names in the same way.
Create a C# program that allows the user to enter his/her 5 friends’ names one by one and display all of them at the last.
Output:Enter the first name
User will enter the name here
Enter the second name
User will enter the name here
.....
....
....
Your friends are
First name
Second name
....
...
Fifth name
Create a C# console application to display family/ friends member’s names in different backgrounds and foreground colors.
Solutions
1: Create a C# program to display your full name on the console window.
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("My name is Nitish Kaushik");
}
}
}
Output:
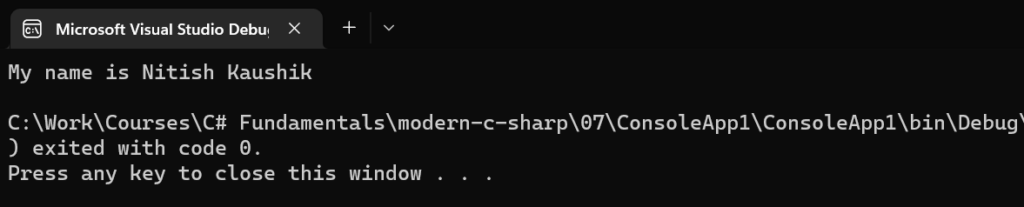
2. Create another C# program to display all the day’s names of the week.
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Sunday");
Console.WriteLine("Monday");
Console.WriteLine("Tuesday");
Console.WriteLine("Wednesday");
Console.WriteLine("Thursday");
Console.WriteLine("Friday");
Console.WriteLine("Saturday");
}
}
}
Output;
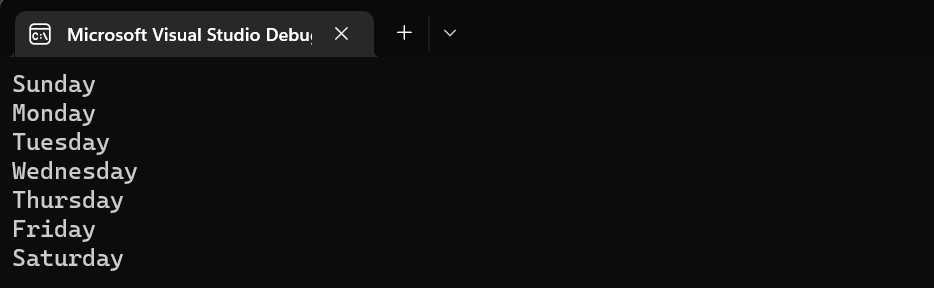
3. Create a C# program that allows the user to enter his/her 5 family member names and display them one by one on the console window.
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter the first name");
var userInput = Console.ReadLine();
Console.WriteLine($"Your first member is {userInput}");
Console.WriteLine("Enter the second name");
userInput = Console.ReadLine();
Console.WriteLine($"Your second member is {userInput}");
Console.WriteLine("Enter the third name");
userInput = Console.ReadLine();
Console.WriteLine($"Your third member is {userInput}");
Console.WriteLine("Enter the fourth name");
userInput = Console.ReadLine();
Console.WriteLine($"Your fourth member is {userInput}");
Console.WriteLine("Enter the fifth name");
userInput = Console.ReadLine();
Console.WriteLine($"Your fifth member is {userInput}");
}
}
}
Output:
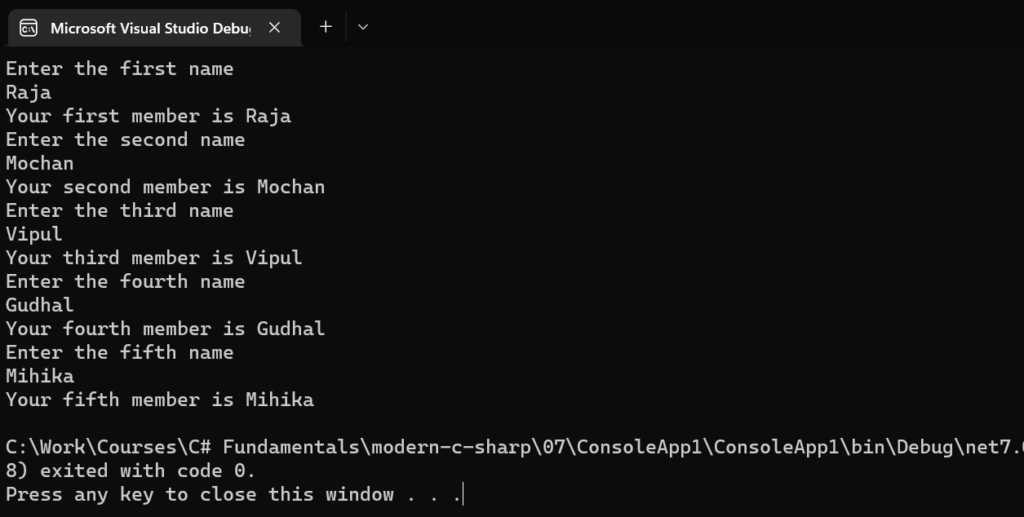
5. Create a C# program that allows the user to enter his/her 5 friends’ names one by one and display all of them at the last.
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter the first name");
var firstName = Console.ReadLine();
Console.WriteLine("Enter the second name");
var secondName = Console.ReadLine();
Console.WriteLine("Enter the third name");
var thirdName = Console.ReadLine();
Console.WriteLine("Enter the fourth name");
var fourthName = Console.ReadLine();
Console.WriteLine("Enter the fifth name");
var fifthName = Console.ReadLine();
Console.WriteLine("Your friends are:");
Console.WriteLine($"First friend is {firstName}");
Console.WriteLine($"Second friend is {secondName}");
Console.WriteLine($"Thied friend is {thirdName}");
Console.WriteLine($"Fourth friend is {fourthName}");
Console.WriteLine($"Fifth friend is {fifthName}");
}
}
}
Output:
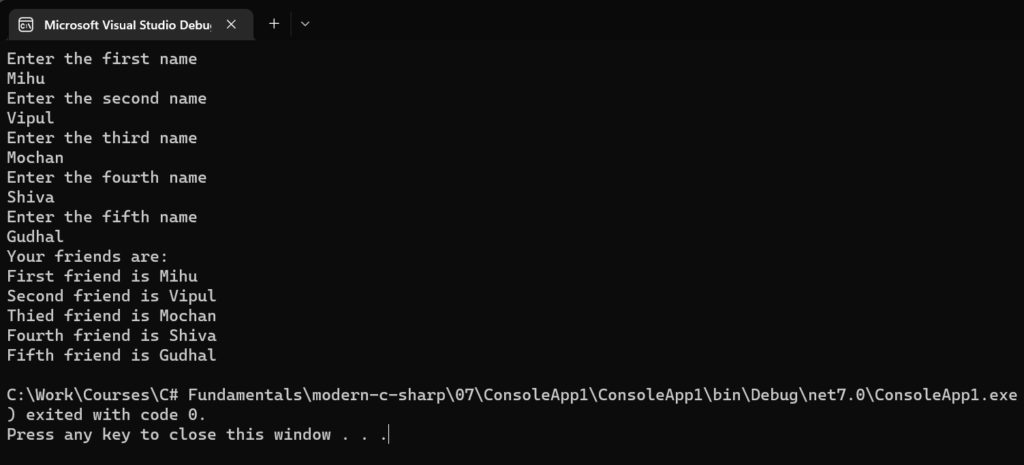
5: Create a C# console application to display family/ friends member’s names in different backgrounds and foreground colors.
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter the first name");
var firstName = Console.ReadLine();
Console.WriteLine("Enter the second name");
var secondName = Console.ReadLine();
Console.WriteLine("Enter the third name");
var thirdName = Console.ReadLine();
Console.WriteLine("Enter the fourth name");
var fourthName = Console.ReadLine();
Console.WriteLine("Enter the fifth name");
var fifthName = Console.ReadLine();
Console.WriteLine("Your friends are:");
Console.BackgroundColor= ConsoleColor.Green;
Console.ForegroundColor= ConsoleColor.Blue;
Console.WriteLine($"First friend is {firstName}");
Console.BackgroundColor = ConsoleColor.Blue;
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine($"Second friend is {secondName}");
Console.BackgroundColor = ConsoleColor.Yellow;
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine($"Thied friend is {thirdName}");
Console.BackgroundColor = ConsoleColor.Red;
Console.ForegroundColor = ConsoleColor.Yellow;
Console.WriteLine($"Fourth friend is {fourthName}");
Console.BackgroundColor = ConsoleColor.Magenta;
Console.ForegroundColor = ConsoleColor.Cyan;
Console.WriteLine($"Fifth friend is {fifthName}");
Console.ResetColor();
}
}
}
Output:
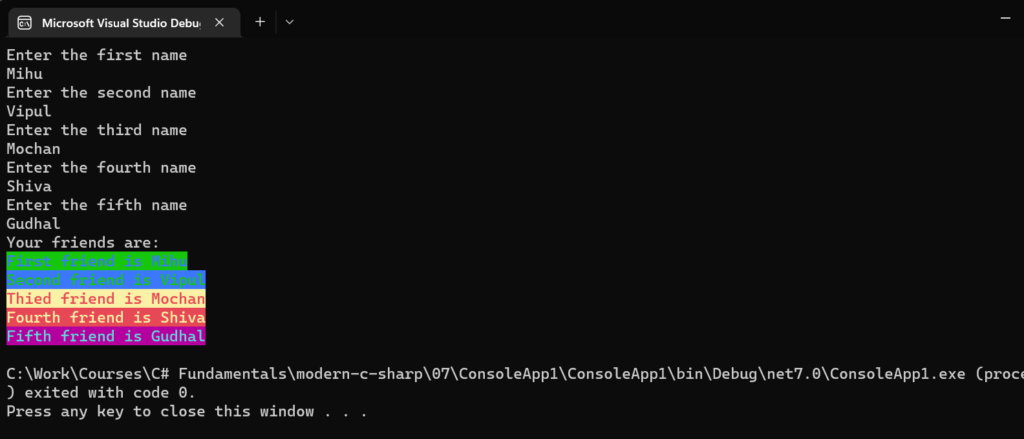