Model in Asp.Net core
Model in asp.net core is one of the main building blocks among the three of building blocks. Models are generally used to deal with the data in the asp.net core application.
What you will learn:
- What is model?
- What is the role of a model?
- How to add a new model in asp.net core application?
- How to return model from action method?
What is model in asp.net core
- A model is a class with .cs (for C#) extension.
- We generally have properties (one for each field of data) in a model class.
What is the role of model in asp.net core
- Model in asp.net core is responsible for the data. It means, if we need to pass some data from one place to other (like controller to view, view to controller, controller to another controller etc.) then we use model for this data transfer.
- We get/set data from/to a source (example: database, in-memory, cloud, etc.) using model.
How to add a new model in asp.net core
If you are using the default folder structure of asp.net core web application then you can add the models in the Models
folder. This Models
folder as available at the root level of the application.
If the Models folder does not exist at the root level of application then feel free to create a new one.
Click here to learn about controllers in asp.net core.
Following the default folder structure of asp.net core application is not mandatory. You can customize the structure as per the need of your own application. For example:
- You can rename the
Models
folder with any other name. - You can create multiple folders inside the
Models
folder based on the features and add the Models classes in these folders. - You can suffix the models by
Model
keyword. example –BookModel.cs
- Create a new class-library type project and create all the models in that class library project.
Now, let’s add a new model by following the default folder structure.
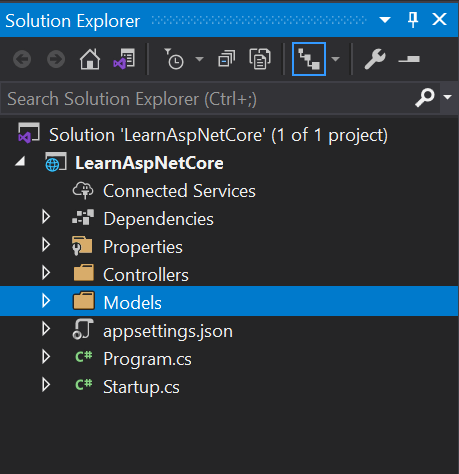
Let’s give the model a name BookModel
and add some properties.
namespace LearnAspNetCore.Models
{
public class BookModel
{
public int Id { get; set; }
public string Title { get; set; }
public string Author { get; set; }
}
}
Remember, A model is used for data in asp.net core web application. Since as of now we do not have any data-dource (database), lets add some in-memory data to understand the concept of models.
Create In-Memory sample data
Let’s add a new folder with name Repository
at the root level and add a new class BookRepository
in this folder.
We will follow the
Repository Pattern
later in this tutorial.
using LearnAspNetCore.Models;
using System.Collections.Generic;
using System.Linq;
namespace LearnAspNetCore.Repository
{
public class BookRepository
{
public List<BookModel> GetAllBooks()
{
return DataSource();
}
public BookModel GetBookById(int id)
{
return DataSource().FirstOrDefault(x=> x.Id == id);
}
public List<BookModel> SearchBooks(string title, string authorName)
{
return DataSource()
.Where(x=> x.Title.Contains(title) || x.Author.Contains(authorName))
.ToList();
}
private List<BookModel> DataSource()
{
return new List<BookModel>()
{
new BookModel(){Id = 1, Title = "MVC", Author = "Nitish" },
new BookModel(){Id = 2, Title = "MVC Core", Author = "Nitish" },
new BookModel(){Id = 3, Title = "C#", Author = "Monika" },
new BookModel(){Id = 4, Title = "Java", Author = "Monika" },
new BookModel(){Id = 5, Title = "PHP", Author = "WebGentle" }
};
}
}
}
Now we can use this BookRepository
class in our controller class.
This is how our BookController class looks like
using Microsoft.AspNetCore.Mvc;
namespace LearnAspNetCore.Controllers
{
public class BookController : Controller
{
public IActionResult Index()
{
return View();
}
public IActionResult GetBook(int id)
{
// get book details from DB or other resources from DB based on the book id.
return View();
}
public IActionResult GetBooks(string bookName, string authorName)
{
// get book details from DB or other resources from DB based on the bookName and/or authorName.
return View();
}
}
}
Let’s use the BookRepository in BookController.
We will create the instance of
BookRepository
by using the new keyword. This will be replaced by dependency injection in upcoming posts.
using LearnAspNetCore.Models;
using LearnAspNetCore.Repository;
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
namespace LearnAspNetCore.Controllers
{
public class BookController : Controller
{
private readonly BookRepository _bookRepository;
public BookController()
{
_bookRepository = new BookRepository();
}
public List<BookModel> GetAllBooks()
{
return _bookRepository.GetAllBooks();
}
public BookModel GetBookById(int id)
{
return _bookRepository.GetBookById(id);
}
public List<BookModel> SearchBook(string bookName, string authorName)
{
return _bookRepository.SearchBooks(bookName, authorName);
}
}
}
Time to test the data using URL
Run the web application by pressing the f5
button from your keyboard (if using visual studio) or run dotnet run
command in CLI.
Once the application is running, enter the following URL in the browser.
GetAllBooks:
https://localhost:44300/book/GetAllBooks
Output:
[
{
"id":1,
"title":"MVC",
"author":"Nitish"
},
{
"id":2,
"title":"MVC Core",
"author":"Nitish"
},
{
"id":3,
"title":"C#",
"author":"Monika"
},
{
"id":4,
"title":"Java",
"author":"Monika"
},
{
"id":5,
"title":"PHP",
"author":"WebGentle"
}
]
GetBookById:
https://localhost:44300/book/GetBookById/1
Output:
{
"id":1,
"title":"MVC",
"author":"Nitish"
}
SearchBook:
https://localhost:44300/book/SearchBook?bookName=Java&authorName=Nitish
Output:
[
{
"id":1,
"title":"MVC",
"author":"Nitish"
},
{
"id":2,
"title":"MVC Core",
"author":"Nitish"
},
{
"id":4,
"title":"Java",
"author":"Monika"
}
]