How controller finds a view in Asp.Net Core
Why do we create all the views inside Views
folder? What if I want to create a view at some other location? How controller finds a view in asp.net core.
These are some questions that come in mind while working on asp.net core mvc application.
In this post we will cover –
- What is View discovery?
- How the application finds a view in asp.net core?
- How to return the view with some other name than the action method’s name?
- How to return a model with the view?
- What if we change the location of view files in asp.net core?
- Who is responsible for view discovery?
What is View discovery?
- When we return a view from action method of asp.net core application then the view discovery takes place.
- View discovery in asp.net core determines which file will be used for a particular action method.
How the controller finds a view in asp.net core?
By default, the application will look for view file at the following locations.
Views/[ControllerName]/[ViewName].cshtml
Views/Shared/[ViewName].cshtml
You might encounter this question often in the interviews –
Why the asp.net core mvc application look for views only in the
Views
folder orShared
folder. Will it work if instead of using Shared, we use Common or something else.
Where this setting is written? Can we override it?
And the answer for the above question is – These settings are written inside the view engine. And in case we want to customize these settings then we need to create our own view engine and use it in the web application.
To return the view from action method we use View()
method.
Use View()
method without passing any parameter –
If we are not passing anything in the View()
method then by default application will search for the view file with corresponding action method name. Let’s say in the above example the action method name is Index
and we are not passing any other name in the View method. So, In this scenario the application will look for the Index.cshtml
file.
public IActionResult Index()
{
return View();
}
How to return the view with some other name than the action method’s name?
On the other hand, if we want to return a view with some other name than the action method name then we need to pass that name in the View method.
Let’s say from this Index
action method, we want to return About.cshtml
view then we need to pass the About
in the View
method.
public IActionResult Index()
{
return View("About");
}
How to return a model with the view?
To return a model with the view in asp.net core we need to pass the object of model in the View()
method.
Pass model without view name –
To pass the model without using the view name, simply pass the object name in the View() method.
public IActionResult Index()
{
var model = new Book();
return View(model);
}
Pass model along with a view name –
To pass the model along with the view name we need to pass the model object as a second parameter in the View() method.
public IActionResult Index()
{
var model = new Book();
return View("About", model);
}
What if we change the location of view files in asp.net core?
If we want to return the view file from some other location then we have following two options.
- Full path
- Relative path
Just for the demo, let’s create a new folder TempView
at the root level of the application and create a view with any name (let’s say) MyTempView.cshtml
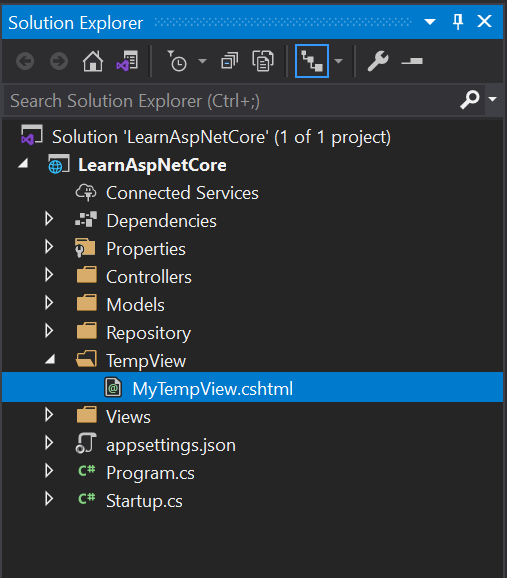
Return the view from other location using full path –
public IActionResult Index()
{
return View("~/TempView/MyTempView.cshtml");
}
Return the view from other location using relative path –
public IActionResult Index()
{
return View("../../TempView/MyTempView");
}
Note: There is no need to pass the view file extension while using the relative path.
Who is responsible for view discovery?
View engine is responsible for the view discovery in the asp.net core application.
By default, the asp.net core web application uses the Razor View Engine.